Next 15, what's new ?
created at 29/08/2024 •updated at 09/12/2024
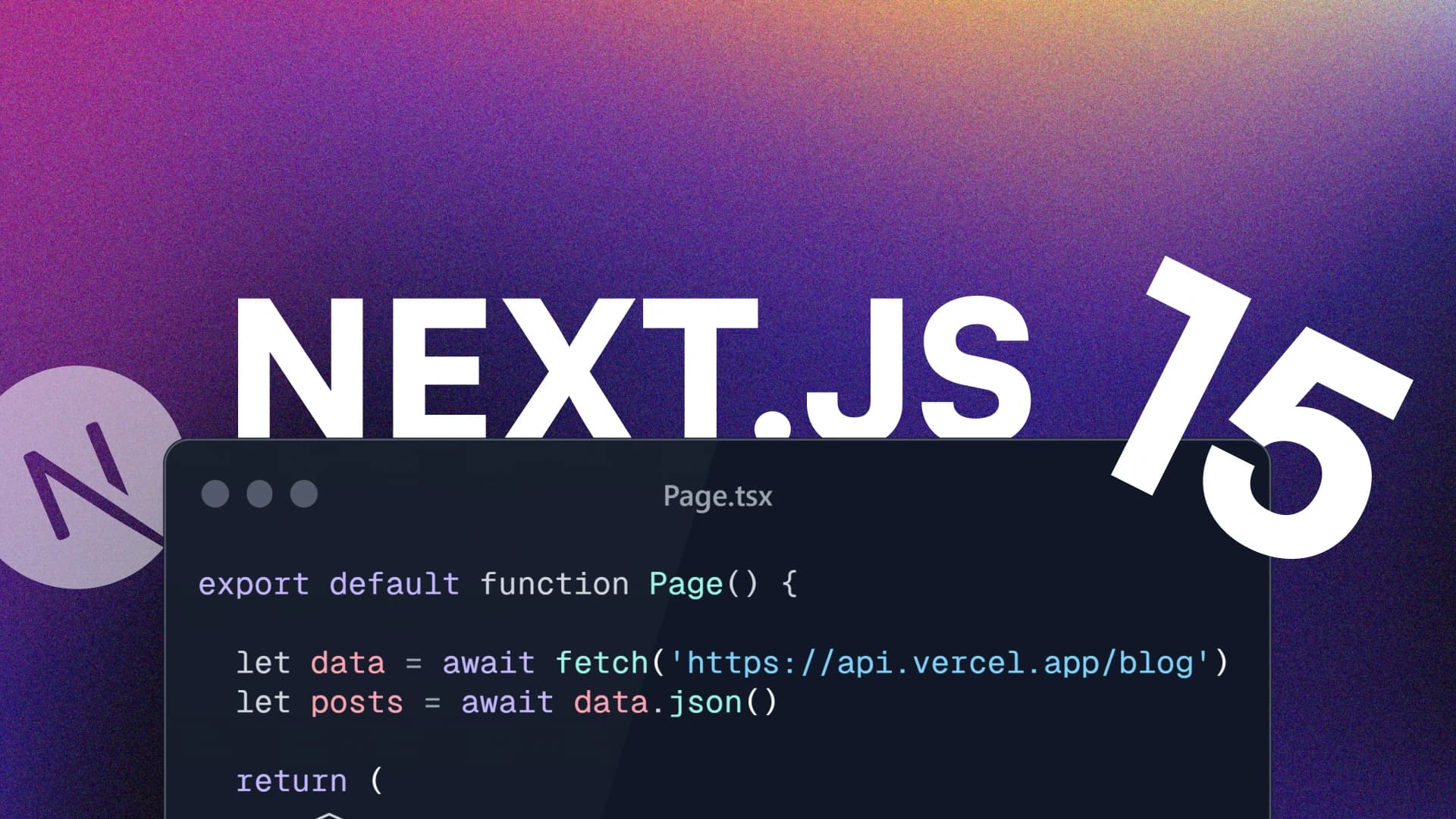
Next.js has continued to evolve as a powerful framework for building modern web applications. With the release of version 15, we see a range of new features, performance improvements, and developer experience enhancements. Whether you’re a seasoned Next.js developer or new to the framework, Next 15 introduces several updates that will improve how you build and scale your applications. Let's dive into the most important updates in Next 15.
Server Actions: Simplified Data Fetching
One of the most exciting features in Next.js 15 is the introduction of Server Actions. Server Actions allow you to perform data mutations directly from your server without the need for API routes. This simplifies data fetching and state management by directly handling the data on the server side.
Example of Server Action
// pages/api/submit.jsexport async function submitData(formData) {'use server'; // This directive tells Next.js to run this function on the server// Handle the form data on the serverconst result = await fetch('https://example.com/api/data', {method: 'POST',body: JSON.stringify(formData),});return result.json();}
This enables cleaner code and allows you to handle form submissions or any server-side logic more seamlessly.
Improved App Router and Routing Patterns
Next.js 15 introduces nested routing and improved dynamic routes, giving developers more flexibility when it comes to structuring their applications. The new app router allows you to create layouts and organize your pages with more control over loading states, parallel rendering, and error boundaries.
Example of Nested Routes
// app/dashboard/layout.jsexport default function DashboardLayout({ children }) {return (<div><Sidebar /><main>{children}</main></div>);}// app/dashboard/settings/page.jsexport default function SettingsPage() {return <h1>Settings</h1>;}// app/dashboard/profile/page.jsexport default function ProfilePage() {return <h1>Profile</h1>;}
With this new pattern, it’s easier to build complex user interfaces with shared layouts without duplicating code.
Turbopack: Lightning-Fast Development Builds
In Next.js 15, Turbopack is now the default bundler in development mode. Turbopack, introduced as an experimental feature in Next.js 13, has been greatly improved and is now more stable. It promises builds that are 10x faster than Webpack, reducing development compile times drastically, especially for large applications.
Configuration Example
No additional configuration is required! Simply update your Next.js version, and Turbopack will be enabled automatically in development.
For larger projects, this can significantly reduce build times and allow developers to iterate faster.
Optimized Static Imports
Next.js 15 improves how static assets like images, fonts, and other files are imported and managed. Static imports are now optimized to only include what's necessary for the initial page load, reducing load times and improving performance metrics such as First Contentful Paint (FCP) and Largest Contentful Paint (LCP).
Example of Optimized Image Import
import Image from 'next/image';export default function Home() {return (<div><Image src="/images/banner.jpg" alt="Banner" width={800} height={400} /></div>);}
With optimized static imports, images are resized and served efficiently, ensuring faster loading times for users.
React Server Components: Better Performance and SEO
Another key highlight of Next.js 15 is the expanded support for React Server Components (RSC). By rendering components entirely on the server, you can significantly reduce client-side JavaScript, improving both performance and SEO.
Server components can fetch data on the server and send the fully-rendered HTML to the client, reducing client-side work.
Example of React Server Component
// components/ProductList.server.jsexport default async function ProductList() {const res = await fetch('https://api.example.com/products');const products = await res.json();return (<ul>{products.map((product) => (<li key={product.id}>{product.name}</li>))}</ul>);}
This approach makes your application lighter, reducing the JavaScript bundle size and improving the user experience.
Conclusion
Next.js 15 brings a wealth of new features and improvements, from Server Actions to Turbopack and expanded support for React Server Components. These updates enhance the performance, scalability, and developer experience of building web applications with Next.js. Whether you’re focusing on improving build times or want better control over routing, Next 15 is a major step forward for the framework.
Be sure to explore these features, update your existing projects, and take full advantage of the new tools that Next.js 15 has to offer!