A Comprehensive Guide to React’s Most Useful Hooks
created at 09/08/2024 •updated at 22/10/2024
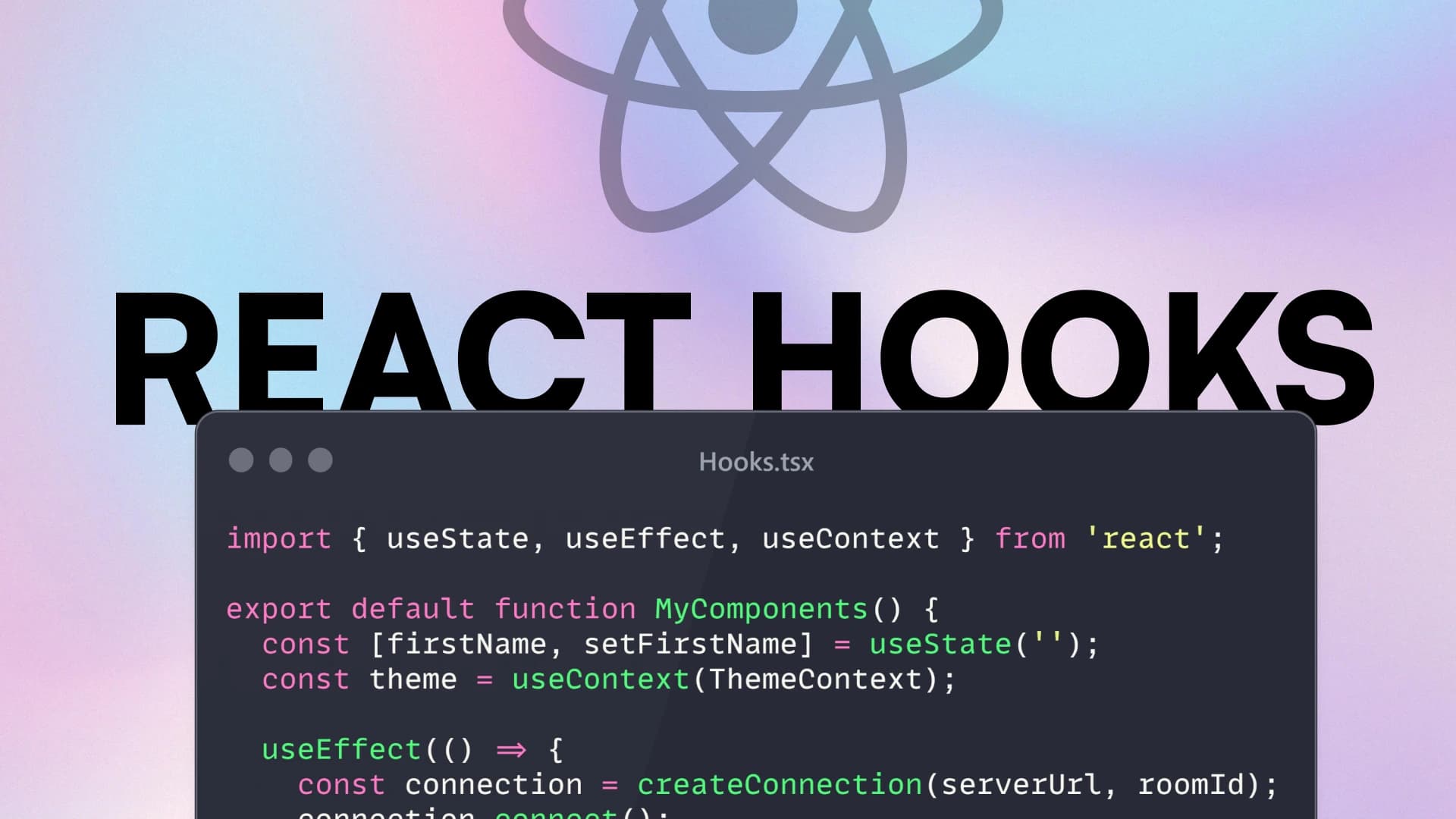
React is one of the most popular JavaScript libraries for building user interfaces, and its hooks feature has revolutionized how developers manage state, side effects, and other functionality in functional components. If you're new to React or looking to deepen your understanding, this guide will walk you through the most useful hooks and how to use them effectively. Let’s dive in!
What Are Hooks?
Before we get into specific hooks, let’s quickly cover what they are. Hooks are functions that let you use state and other React features without writing a class. They provide a way to “hook into” React’s ecosystem, allowing you to manage component state, side effects, context, and much more—all within functional components.
1. useState: Managing Local State
One of the most commonly used hooks is useState
, which allows you to add state to functional components.
How it works:
useState
takes an initial state value and returns an array with two elements: the current state and a function to update that state.
Example:
import React, { useState } from 'react';function Counter() {const [count, setCount] = useState(0);return (<div><p>Current Count: {count}</p><button onClick={() => setCount(count + 1)}>Increment</button></div>);}export default Counter;
In this example, the count
variable holds the current state, and setCount
is the function that updates it when the button is clicked.
2. useEffect: Handling Side Effects
The useEffect
hook is essential for handling side effects in your component, such as fetching data, subscribing to events, or manually manipulating the DOM.
How it works:
useEffect
accepts a function that runs after every render. You can also provide a dependency array to control when the effect should re-run.
Example:
import React, { useState, useEffect } from 'react';function DataFetcher() {const [data, setData] = useState(null);useEffect(() => {fetch('https://api.example.com/data').then(response => response.json()).then(data => setData(data));}, []); // Empty array means this effect runs once after the initial render.return (<div><p>Data: {data ? JSON.stringify(data) : 'Loading...'}</p></div>);}export default DataFetcher;
Here, the useEffect
hook fetches data from an API when the component is first rendered. The empty dependency array ([]
) ensures the fetch only happens once.
3. useContext: Sharing State Across Components
The useContext
hook allows you to access global data without having to pass props through every level of your component tree. It works in conjunction with React's Context
API.
How it works:
You create a context using React.createContext()
, and then access the context’s value inside your component using useContext
.
Example:
import React, { createContext, useContext } from 'react';// Create a contextconst UserContext = createContext();function DisplayUser() {const user = useContext(UserContext);return <p>User: {user.name}</p>;}function App() {const user = { name: 'John Doe' };return (<UserContext.Provider value={user}><DisplayUser /></UserContext.Provider>);}export default App;
In this example, the DisplayUser
component consumes the user
object from the UserContext
, avoiding the need to pass props directly.
4. useReducer: More Complex State Logic
When your state logic becomes more complex (such as multiple sub-values or actions), useReducer
can be a better alternative to useState
.
How it works:
useReducer
accepts a reducer function and an initial state, returning the current state and a dispatch function. The reducer function determines how to update the state based on the action.
Example:
import React, { useReducer } from 'react';function reducer(state, action) {switch (action.type) {case 'increment':return { count: state.count + 1 };case 'decrement':return { count: state.count - 1 };default:return state;}}function Counter() {const [state, dispatch] = useReducer(reducer, { count: 0 });return (<div><p>Count: {state.count}</p><button onClick={() => dispatch({ type: 'increment' })}>+</button><button onClick={() => dispatch({ type: 'decrement' })}>-</button></div>);}export default Counter;
Here, useReducer
manages the state for a counter, allowing you to handle more complex state transitions with a switch statement.
5. useRef: Accessing DOM Elements and Keeping Persistent Values
The useRef
hook is useful when you need to access DOM elements directly or store a value that persists across renders but doesn't cause a re-render when updated.
How it works:
useRef
returns a mutable object that holds a .current
property. You can use this property to store values.
Example:
import React, { useRef } from 'react';function TextInputFocus() {const inputRef = useRef(null);const focusInput = () => {inputRef.current.focus();};return (<div><input ref={inputRef} type="text" /><button onClick={focusInput}>Focus Input</button></div>);}export default TextInputFocus;
In this example, the inputRef
is assigned to the input element, allowing the button to programmatically focus the input field when clicked.
6. useMemo: Optimizing Performance
The useMemo
hook is used to optimize performance by memoizing the result of a function that is computationally expensive. It will recompute the value only when one of its dependencies changes.
How it works:
useMemo
takes two arguments: a function that returns a value, and a dependency array. The function will only re-run when one of the dependencies changes.
Example:
import React, { useState, useMemo } from 'react';function ExpensiveCalculation(num) {console.log('Calculating...');return num * 2;}function MemoizedComponent() {const [count, setCount] = useState(0);const [otherCount, setOtherCount] = useState(0);const memoizedValue = useMemo(() => ExpensiveCalculation(count), [count]);return (<div><p>Count: {count}</p><p>Memoized Calculation: {memoizedValue}</p><p>Other Count: {otherCount}</p><button onClick={() => setCount(count + 1)}>Increment Count</button><button onClick={() => setOtherCount(otherCount + 1)}>Increment Other Count</button></div>);}export default MemoizedComponent;
In this example, the ExpensiveCalculation
function only runs when count
changes, thanks to useMemo
. This can help prevent unnecessary re-computation and improve performance.
Conclusion
React hooks offer a powerful and flexible way to manage state, side effects, and more in functional components. Whether you’re managing simple state with useState
or optimizing performance with useMemo
, these hooks provide an intuitive API to help you build dynamic and responsive UIs.
With these core hooks in your toolkit, you're well on your way to building more efficient and maintainable React applications. Happy coding!