Mastering Arrays in JavaScript
created at 29/08/2024 •updated at 13/12/2024
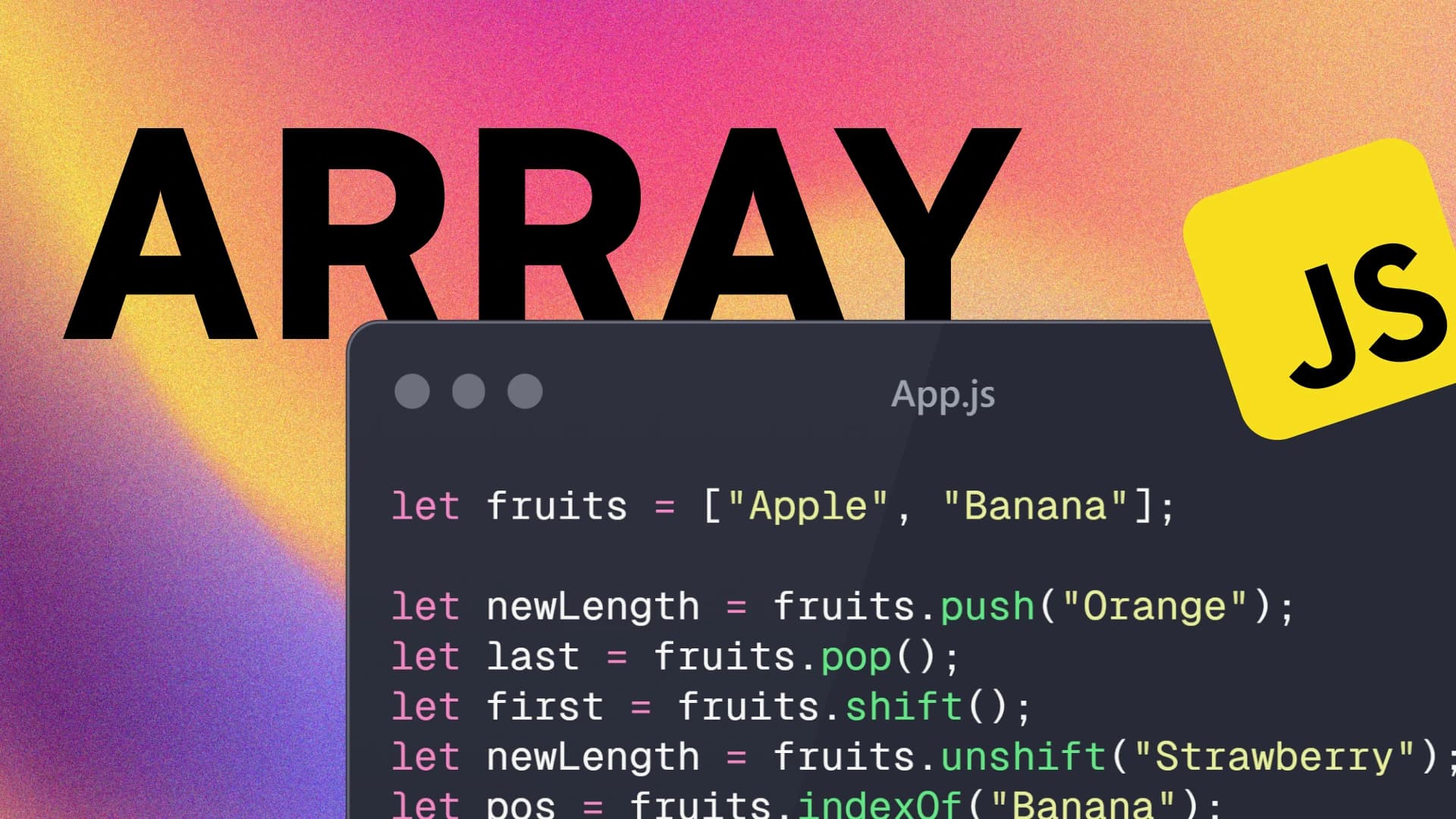
Arrays are one of the most fundamental data structures in JavaScript. They allow you to store, manage, and manipulate a collection of values, whether those values are numbers, strings, or even objects. Understanding how to work with arrays effectively is essential for anyone looking to improve their JavaScript skills. In this article, we’ll dive into the basics of arrays and explore the various methods and functions available for working with them.
What is an Array?
An array is a special type of object in JavaScript that allows you to store multiple values in a single variable. Each value in an array is stored in a specific order and can be accessed by its index (starting from 0).
const fruits = ['apple', 'banana', 'cherry'];console.log(fruits[0]); //>_ appleconsole.log(fruits[1]); //>_ bananaconsole.log(fruits[2]); //>_ cherry
Creating Arrays
You can create arrays using two main methods:
Array Literal: This is the most common way to create arrays.
javascriptCopier le codeconst numbers = [1, 2, 3, 4, 5];
Array Constructor: You can also create an array using the
Array()
constructor, though this method is less common.javascriptCopier le codeconst moreNumbers = new Array(6, 7, 8, 9);
Adding and Removing Elements
push(
) andpop()
To add elements to the end of an array, you can use the push()
method. Conversely, to remove elements from the end, use pop()
.
const animals = ['cat', 'dog'];animals.push('elephant');console.log(animals); //>_ ['cat', 'dog', 'elephant']animals.pop();console.log(animals); //>_ ['cat', 'dog']
2. unshift()
and shift()
If you need to add or remove elements from the beginning of an array, use unshift()
to add and shift()
to remove.
const colors = ['red', 'green', 'blue'];colors.unshift('yellow');console.log(colors); //>_ ['yellow', 'red', 'green', 'blue']colors.shift();console.log(colors); //>_ ['red', 'green', 'blue']
Finding Elements in an Array
indexOf()
and includes()
To find the position of an element in an array, you can use indexOf()
. If the element doesn’t exist, it returns -1
. To check if an element exists in the array, you can use includes()
.
const numbers = [1, 2, 3, 4, 5];console.log(numbers.indexOf(3)); //>_ 2console.log(numbers.includes(6)); //>_ false
Iterating Over Arrays
The classic way to iterate through an array is by using a for
loop.
const cars = ['Tesla', 'BMW', 'Audi'];for (let i = 0; i < cars.length; i++) {console.log(cars[i]);}
A more modern approach to iterate over arrays is by using the forEach()
method.
const fruits = ['apple', 'banana', 'cherry'];fruits.forEach(fruit => {console.log(fruit);});
Transforming Arrays
map()
function
The map()
method creates a new array by applying a function to each element of the original array.
const numbers = [1, 2, 3, 4];const doubled = numbers.map(num => num * 2);console.log(doubled); //>_ [2, 4, 6, 8]
filter()
function :
The filter()
method creates a new array that contains only the elements that pass a specific condition.
const ages = [12, 18, 20, 30, 40];const adults = ages.filter(age => age >= 18);console.log(adults); //>_ [18, 20, 30, 40]
reduce()
function :
The reduce()
method reduces an array to a single value by applying a function that accumulates the results.
const numbers = [1, 2, 3, 4];const sum = numbers.reduce((acc, current) => acc + current, 0);console.log(sum); //>_ 10
Modifying the Array
splice()
function :
The splice()
method can be used to add or remove elements from any position in the array.
const months = ['January', 'March', 'April', 'June'];months.splice(1, 0, 'February');console.log(months); // ['January', 'February', 'March', 'April', 'June']months.splice(3, 1);console.log(months); // ['January', 'February', 'March', 'June']
Combining and Slicing Arrays
concat()
function :
The concat()
method combines two or more arrays into one new array.
const firstArray = [1, 2, 3];const secondArray = [4, 5, 6];const combinedArray = firstArray.concat(secondArray);console.log(combinedArray); //>_ [1, 2, 3, 4, 5, 6]
slice()
function :
The slice()
method returns a shallow copy of a portion of an array. It does not modify the original array.
const letters = ['a', 'b', 'c', 'd', 'e'];const sliced = letters.slice(1, 3);console.log(sliced); //>_ ['b', 'c']
Sorting and Reversing Arrays
sort()
function :
The sort()
method sorts the elements of an array in place.
const numbers = [40, 10, 30, 20];numbers.sort((a, b) => a - b); // Sorts in ascending orderconsole.log(numbers); //>_ [10, 20, 30, 40]
reverse()
function :
The reverse()
method reverses the elements of an array.
const letters = ['a', 'b', 'c', 'd'];letters.reverse();console.log(letters); //>_ ['d', 'c', 'b', 'a']
Conclusion
Arrays are a powerful and versatile tool in JavaScript. From adding and removing elements to transforming and combining arrays, mastering these methods and functions will allow you to manipulate data effectively. By practicing these array methods, you'll become more confident in handling complex data structures in your JavaScript applications.
Keep experimenting, and happy coding!