How to Learn React: A Beginner's Guide
created at 16/07/2024 •updated at 09/12/2024
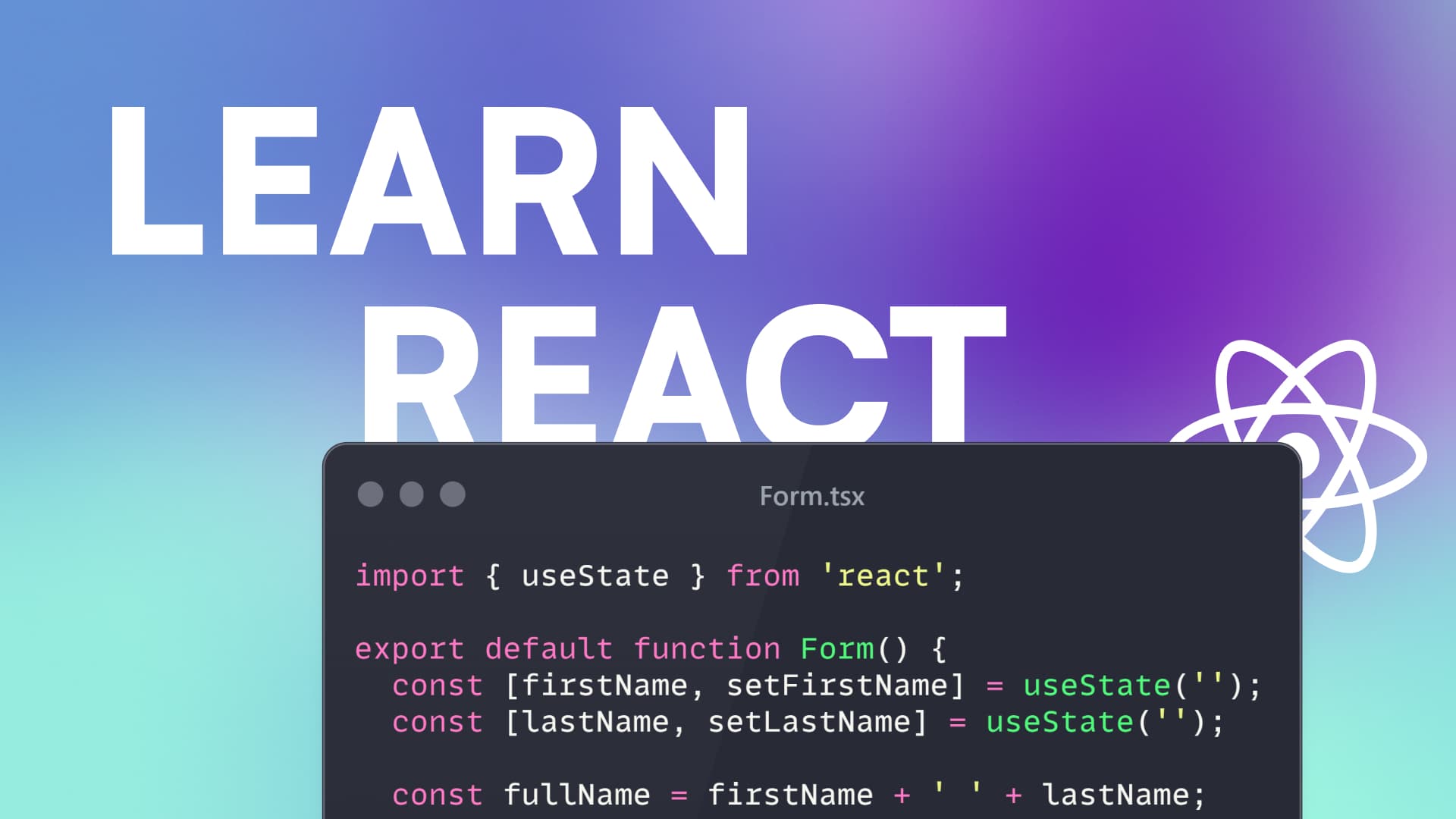
React has become one of the most popular JavaScript libraries for building user interfaces. Whether you’re developing a website, a web app, or even a mobile app, React is a powerful tool that allows you to create dynamic and interactive UIs with ease. In this guide, we'll cover the essential steps you need to take to get started with React.
What is React?
React is an open-source JavaScript library developed by Meta. It’s used to build user interfaces, particularly for single-page applications where you need a fast, interactive experience. Unlike traditional methods of manipulating the DOM (Document Object Model), React introduces a more efficient way to update the UI by using a virtual DOM. This approach minimizes the amount of work the browser has to do, leading to faster performance.
In simple terms, React allows you to build components—small, reusable pieces of code that represent a part of the user interface. These components can be as simple as a button or as complex as an entire form. The key to mastering React is understanding how to build and manage these components effectively.
Logic of Components
At the heart of React is the concept of components. A component in React is a self-contained block of code that renders a piece of the UI. Components can be thought of as building blocks that you can combine to create a complete interface.
Components can be either functional or class-based. Functional components are simpler and are the recommended way to build components in modern React. They are just JavaScript functions that return JSX (which we’ll discuss next). Class-based components, on the other hand, use ES6 classes and offer more advanced features but are becoming less common with the introduction of React Hooks.
// functional componentfunction Welcome() {return <h1>Hello, World!</h1>;}// class-based componentclass Welcome extends React.Component {render() {return <h1>Hello, World!</h1>;}}
Understanding JSX
JSX, or JavaScript XML, is a syntax extension for JavaScript that looks a lot like HTML. JSX is used in React to describe what the UI should look like. It might seem strange at first to write HTML-like code inside your JavaScript, but it’s one of the key features that make React so powerful.
With JSX, you can write markup directly within your JavaScript code, which makes it easier to visualize your UI and maintain your code. Under the hood, JSX is converted to regular JavaScript, so it’s fully compatible with the rest of your code.
const element = <h1>Hello, World!</h1>;
JSX allows you to include JavaScript expressions within your markup by using curly braces . This makes it very flexible for creating dynamic user interfaces.
const user = { name: "John" };const element = <h1>Hello, {user.name}!</h1>;
React Hooks
React Hooks are functions that let you use state and other React features in functional components. Before hooks, only class components could manage state and lifecycle events. Hooks introduced in React 16.8, made functional components much more powerful and are now the standard way to work with state and side effects in React.
Common Hooks:
useState: Allows you to add state to a functional component.
useEffect: Lets you perform side effects (like data fetching or subscribing to a service) in your components.
useContext: Provides a way to share values like themes or user data across components without passing props manually at every level.
Exemple of "useState" Hook:
import React, { useState } from 'react';function Counter() {// initializes the state to 0const [count, setCount] = useState(0);return (<div><p>You clicked {count} times</p><button onClick={() => setCount(count + 1)}>Click me</button></div>);}
Passing Props to a Component
Props, short for "properties," are a way of passing data from one component to another. Props are used to pass dynamic data to components, making them more flexible and reusable. In React, props are passed to components in the same way you pass arguments to a function.
When you pass props to a component, they are accessed inside the component using this.props
in class components or directly in functional components.
function Welcome(props) {return <h1>Hello, {props.name}!</h1>;}// Usage<Welcome name="John" />
In the example above, the Welcome
component receives a name
prop and uses it to render a personalized greeting. This makes the component more reusable because you can pass different names without changing the component itself.
Beware of props drilling (occurs when a prop needs to be passed through several layers of nested components to reach a deeply nested child component) Several solutions exist such as the useContext hook or State Management Libraries (an article on Zustand is available right here)
When and How to Use React? Which Framework?
React is incredibly versatile, making it a great choice for a wide range of projects, from simple landing pages to complex web applications. But when should you use React, and which framework should you pair it with?
When to Use React:
Single-Page Applications (SPAs): If your project requires a highly interactive UI where users don't want to wait for new pages to load, React is a great choice.
Reusable Components: If you need to create a lot of similar UI elements that can be reused across your application, React’s component-based architecture will save you a lot of time and effort.
Large-Scale Projects: React's modular nature makes it easy to manage and scale large applications.
Which Framework?
React can be used with various frameworks and tools depending on your project needs:
Next.js: A popular framework for server-side rendering (SSR) and static site generation (SSG) with React. It's a great choice if SEO is a priority.
Gatsby: Another React-based framework, Gatsby is ideal for building fast static websites.
Create React App: If you’re just starting, Create React App is a tool that sets up everything you need to start developing a React application without the hassle of configuration.
React’s flexibility means you can also integrate it with other JavaScript libraries or frameworks if needed.
Conclusion
Learning React might seem overwhelming at first, but by understanding the basics—like components, JSX, hooks, and props—you’ll be well on your way to building powerful and dynamic web applications. Start small, practice regularly, and don’t be afraid to experiment with new concepts. Before you know it, you’ll be building complex user interfaces with ease!