Begin Next js
created at 29/08/2024 •updated at 09/12/2024
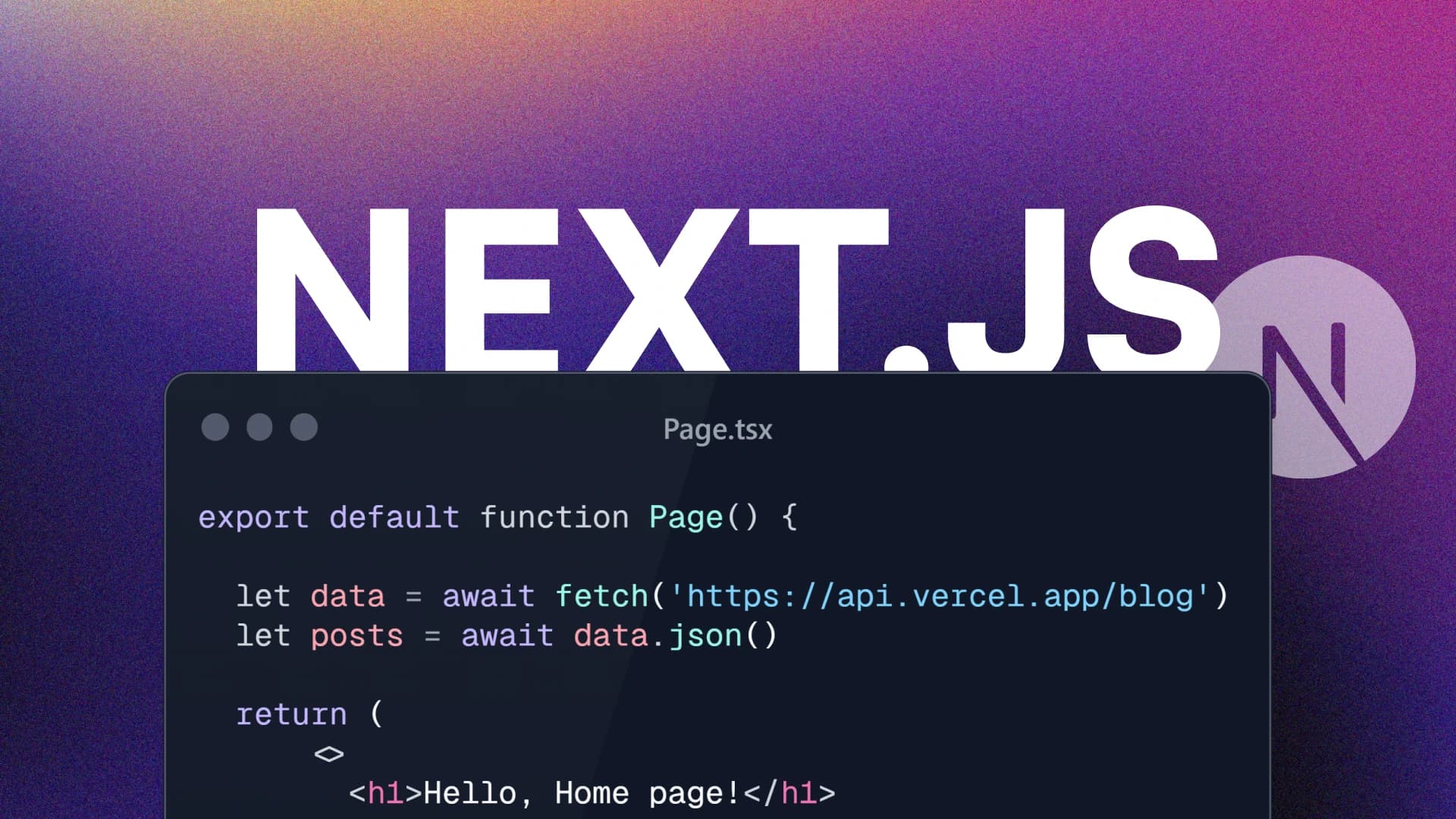
Next.js has emerged as a popular choice for building modern web applications due to its flexibility, performance optimizations, and ease of use. If you're looking to become proficient in Next.js, there are specific areas you need to focus on. In this guide, we will walk through the fundamental steps and elements you should learn to master the framework.
1. Understand the Basics of React
Before diving deep into Next.js, it's important to have a solid foundation in React, as Next.js is built on top of React. Key concepts include:
Components: Learn how to create functional and class components.
JSX Syntax: Understand how to use JSX, which allows you to write HTML-like code in your JavaScript files.
Props and State: Learn how to pass data through props and manage state within components.
Lifecycle Methods: Familiarize yourself with hooks like
useEffect
anduseState
for managing side effects and state.
Example of a simple React component:
function Greeting({ name }) {return <h1>Hello, {name}!</h1>;}
Once you're comfortable with these React fundamentals, you'll find it easier to transition to Next.js.
2. Set Up Your First Next.js Project
The first technical step in mastering Next.js is learning how to set up a Next.js project. To get started, use the create-next-app
tool, which scaffolds a new Next.js project for you.
npx create-next-app@latest
After installation, run the development server:
npm run dev
Once you have your project set up and running, open it in your browser at http://localhost:3000
. From here, you can start learning the structure and components of a Next.js application.
3. Master Routing in Next.js
The App Router
Routing in Next.js is file-based, meaning the structure of your directories and files defines the routes of your application. One of the major changes in recent versions of Next.js is the App Router, which provides a more intuitive way to manage routes.
To create a new route, you create folders inside the app
directory and add a page.js
file to define the route:
/app/aboutpage.js
The about/page.js
file will now be accessible at http://localhost:3000/about
. This file will contain the code for your "About" page:
export default function AboutPage() {return <h1>About Us</h1>;}
Dynamic Routing
Next.js also makes it easy to create dynamic routes, such as user profiles or blog posts. For example, to create a route that changes based on a parameter (like a post ID), you can use square brackets in the file name:
/app/posts/[id]page.js
In page.js
, you can access the dynamic id
using the useRouter
hook:
import { useRouter } from 'next/router';export default function PostPage() {const router = useRouter();const { id } = router.query;return <h1>Post ID: {id}</h1>;}
This allows you to create a flexible URL structure while keeping your code clean and organized.
4. Learn About Pre-rendering: SSR vs SSG
One of Next.js's most powerful features is its ability to pre-render pages, improving performance and SEO. You need to understand the difference between Server-Side Rendering (SSR) and Static Site Generation (SSG).
Static Site Generation (SSG)
SSG generates static HTML files at build time, making them highly performant. Pages using SSG can fetch data at build time with getStaticProps
:
export async function getStaticProps() {const res = await fetch('https://api.example.com/data');const data = await res.json();return {props: {data,},};}export default function HomePage({ data }) {return <div>{data.title}</div>;}
Server-Side Rendering (SSR)
With SSR, pages are rendered on each request. You use getServerSideProps
to fetch data at runtime:
export async function getServerSideProps() {const res = await fetch('https://api.example.com/data');const data = await res.json();return {props: {data,},};}export default function HomePage({ data }) {return <div>{data.title}</div>;}
Learning when to use SSG versus SSR is key for optimizing your Next.js applications.
5. Data Fetching and API Routes
In addition to pre-rendering, Next.js makes data fetching and API management simple.
Client-Side Fetching
Sometimes, you'll need to fetch data on the client side. You can do this with the standard fetch
API, typically inside a useEffect
hook:
import { useEffect, useState } from 'react';export default function DataFetchingComponent() {const [data, setData] = useState(null);useEffect(() => {fetch('/api/hello').then((res) => res.json()).then((data) => setData(data));}, []);return <div>{data ? data.message : 'Loading...'}</div>;}
API Routes
Next.js also allows you to build backend API routes directly inside your application. To create an API route, add a file under the /pages/api
directory:
/pages/apihello.js
In hello.js
:
export default function handler(req, res) {res.status(200).json({ message: 'Hello from Next.js API!' });}
You can access this API at http://localhost:3000/api/hello
. Mastering API routes is important if you want to handle server-side logic in your Next.js app.
6. Learn Layouts and Code Splitting
Layouts
Layouts in Next.js allow you to create reusable structures, such as headers and footers, shared across multiple pages. With the new App Router, you can easily define layouts.
For example, in app/layout.js
:
export default function RootLayout({ children }) {return (<html><body><header><h1>My Website</h1></header><main>{children}</main><footer>© 2024 My Website</footer></body></html>);}
This layout will be used across all pages unless otherwise specified.
Code Splitting
Next.js automatically splits your JavaScript bundle so that each page only loads the necessary code. This reduces the amount of code sent to the client, improving performance.
You don't have to do anything special to enable this, but understanding how code splitting works can help when optimizing large applications.
7. Optimize Performance and SEO
Next.js offers several built-in optimizations:
Image Optimization: Use the
next/image
component for automatic image optimization.Link Prefetching: The
next/link
component preloads linked pages in the background, making navigation faster.Meta Tags and SEO: Use the
Head
component to add meta tags and improve your site's SEO:
import Head from 'next/head';export default function SEOPage() {return (<div><Head><title>My SEO-Optimized Page</title><meta name="description" content="This is an SEO-optimized page." /></Head><h1>Hello World!</h1></div>);}
8. Learn Deployment and Hosting
Finally, once your application is ready, you'll need to learn how to deploy it. Fortunately, Next.js integrates seamlessly with platforms like Vercel (the creators of Next.js), Netlify, and AWS. Deploying on Vercel is as simple as connecting your GitHub repository and clicking "Deploy."
Conclusion
Mastering Next.js involves understanding a combination of React fundamentals and Next.js-specific features like routing, pre-rendering, data fetching, and performance optimization. By focusing on the steps outlined above, you'll gain the skills needed to build fast, scalable, and SEO-friendly web applications.
With consistent practice, you'll soon be able to harness the full power of Next.js and use it to develop modern, production-ready web applications.